Python Interview Questions and Answers
4.9 out of 5 based on 2041 votesLast updated on 17th May 2023 3.1K Views
- Bookmark
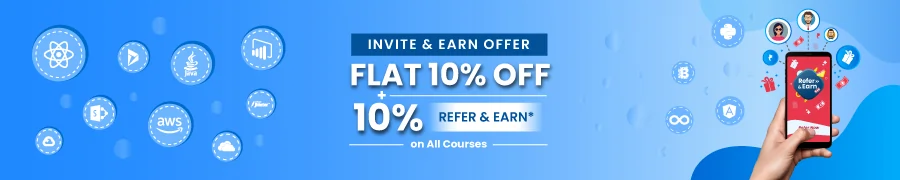
Hi, I'm here today to share something that I hope will bring you value, especially if you're preparing for a Python job interview.
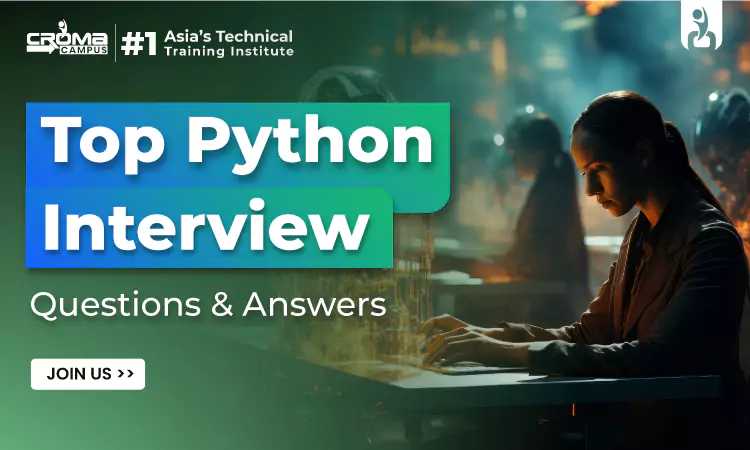
Are you ready to face a Python Interview in 2025? Whether you are a fresher or have some experience, these questions will help you prepare. If you are serious about diving deeper into Python, consider enrolling in an Advanced Python Programming Course. Below, I have provided Python Interview Questions and Answers to help you get ready for your next interview!
Q1. What is memory management in Python?
Answer: Python uses an automatic memory management system with a garbage collector that handles memory allocation and deallocation. The key component is reference counting, and a cyclic garbage collector cleans up any unused objects. If you are preparing for Python Interview Questions for freshers, this topic is essential to understand as it helps in building efficient applications.
Q2. **Explain *args and kwargs in Python.
Answer: The args and kwargs variables in Python allow functions to accept variable amounts of arguments. *args collects positional arguments as a tuple, and **kwargs collects keyword arguments as a dictionary. This feature is especially useful when you don't know in advance how many arguments will be passed to a function.
Q3. What are Python generators and how are they different from regular functions?
Answer: Python generators are a type of iterable, like a list, but instead of holding all values in memory at once, they yield one value at a time using the yield keyword. Regular functions return a value all at once, but generators only produce values when needed. This difference makes generators more memory efficient. If you are aiming for a deeper understanding, enrolling in a Python Course in Gurgaon will help you master generators.
Q4. What is duck typing in Python?
Answer: Duck typing is a concept in Python where the type or class of an object is determined by its behavior, not by its inheritance. If an object has the necessary methods or behaviors, Python considers it to be of that type, even if it’s not explicitly declared as such. Understanding duck typing is crucial for flexible and dynamic programming in Python. A Python Online Course can help you grasp this concept more thoroughly.
Q5. How does exception handling work in Python?
Answer: Python uses try, except, else, and finally blocks for exception handling. Code that might raise an exception is placed inside the try block. If an exception occurs, it’s caught by the except block. The else block runs if no exception is raised, and the finally block always executes, ensuring resource cleanup.
Q6. What is the Global Interpreter Lock (GIL) in Python?
Answer: The Global Interpreter Lock (GIL) is a mutex that allows only one thread to execute Python bytecode at a time. This means that Python is not well-suited for CPU-bound multi-threaded programs. However, for I/O-bound tasks, threading can still be useful.
Q7. What is a context manager in Python?
Answer: A context manager is used in Python to handle resource management (like opening and closing files). The context manager defines __enter__ and __exit__ methods and is typically used with the with keyword. It’s an essential concept in Python programming, especially if you plan to work with files or network connections.
Q8. What is the yield keyword in Python?
Answer: The yield creates a generator. When a function contains yield, it returns a generator instead of a single value. This function can produce a sequence of values over time, which makes it memory efficient. Learning about yield and how it compares to regular functions is key when preparing for Python Interview Questions for freshers.
Q9. What is the difference between str and repr?
Answer: In Python, __str__ is used to provide a human-readable string representation of an object, while __repr__ provides an unambiguous string representation. The purpose of __repr__ is to provide a string that can be used to recreate the object. Understanding these methods will help you in debugging and building better Python applications.
Q10. What is the zip function in Python?
Answer: The zip () function in Python takes multiple iterables and aggregates them into a single iterator of tuples. Each tuple contains elements from each iterable at the corresponding position. This is useful when you need to iterate over two or more lists in parallel.
Q11. What are Lambda functions in Python?
Answer: A lambda function typically used for short, one-off operations. Lambda functions are beneficial when you need a quick function but don't want to define a full function using def. This concept is common in Python, and being able to apply it effectively will help you solve problems faster in interviews.
Q12. What are list comprehensions in Python?
Answer: They allow you to write compact code for creating lists based on existing lists or iterables. For example, you can filter or transform data in a list in a single line. This is a useful feature that saves time and makes code more readable.
Q13. What is a decorator in Python?
Answer: A decorator is used for adding functionality to an existing function without changing its structure. @decorator syntax is commonly used in Python. Understanding how to create and use decorators is a common question in Python interviews. By taking a Python Certification Course, you can gain practical experience in writing and applying decorators.
Q14. Deep copy versus shallow copy: what's the difference?
Answer: A shallow copy of an object creates a new object, but doesn’t recursively copy nested objects. This distinction is important for understanding how to work with complex data structures.
Q15. What is multithreading in Python?
Answer: Multithreading in Python allows multiple threads to run concurrently, which can improve performance, especially for I/O-bound tasks. However, due to the Global Interpreter Lock (GIL), multithreading does not improve performance for CPU-bound tasks. For Python development, understanding multithreading is crucial when working with large applications.
Q16. Can you explain the difference between the Python functions is and ==?
Answer: The == operator checks if the values of two objects are equal, whereas the is operator checks if two objects reference the same memory location. This distinction is crucial when comparing objects in Python. For more clarity on this topic, taking an Advanced Python Programming Course will help you understand the nuances of object comparison in Python.
Q17. What is a Python module?
Answer: Modules allow you to organize and reuse code in a structured way. You can import a module in any Python script using the import keyword. If you are preparing for Python Interview Questions for freshers, understanding the concept of modules is essential. Join a Python Course in Delhi to learn how to effectively use and create Python modules.
Q18. What are the differences between lists and tuples in Python?
Answer: A list in Python is mutable, meaning you can change its elements, while a tuple is immutable. This immutability feature of tuples makes them useful in situations where data should not be modified. Lists are more versatile but come with additional overhead. For freshers, learning the distinctions between lists and tuples is important when dealing with collections in Python.
Q19. How can you handle missing values in a list or data structure?
Answer: In Python, missing values can be handled by using None, which represents a null value. You can also use libraries like pandas for more advanced handling, such as replacing missing values with a default or calculated value.
Q20. What is the self-keyword in Python?
Answer: The self-keyword is used in Python to represent an instance of a class. It allows access to the instance’s attributes and methods from within the class. When defining methods in a class, you must include self as the first parameter. This is a foundational concept in object-oriented programming with Python.
You May Also Read:
Data Science Interview Questions and Answers
Q21. What is the __init__ method in Python?
Answer: The __init__ method is a special method in Python used to initialize newly created objects. It is the constructor method that is automatically called when an object of a class is instantiated. Understanding how to use the __init__ method is key to creating and managing classes.
Q22. Pass statements in Python serve what purpose?
Answer: It is useful when you need to create an empty block of code, such as in a function or class, but you haven’t implemented it yet. Learning the uses of pass is important for writing clean and concise code.
Q23. What are the built-in data types in Python?
Answer: Python has several built-in data types including integers (int), floating point numbers (float), strings (str), lists (list), tuples (tuple), dictionaries (dict), and sets (set). These data types are fundamental to Python programming, and understanding them is essential for any beginner.
Q24. What is the use of sorted() in Python?
Answer: The sorted() function in Python returns a new sorted list from the elements of any iterable (like lists, tuples, or dictionaries). It doesn’t modify the original iterable but instead creates a new one. If you are preparing for Python Interview Questions for freshers, understanding sorting operations in Python will help you with coding challenges.
Q25. How can you convert a string to an integer in Python?
Answer: In Python, a string can be converted to an integer with the int() function. If the string is not a valid integer, Python will raise a ValueError. This is a common task when working with user input.
Q26. How do you create a class in Python?
Answer: Using the class keyword and the class name, create a class in Python. Inside the class, you define methods and attributes. A class is a blueprint for creating objects, and learning how to create and work with classes is foundational for object-oriented programming.
Q27. What is the difference between del and remove() in Python?
Answer: The del keyword is used to delete a variable or an element at a specific index in a list, while the remove() method is used to remove the first occurrence of a value in a list. Understanding these differences will help you manage and manipulate data structures efficiently.
Q28. What is a Python iterator?
Answer: Python provides an iterator interface with the methods __iter__() and __next__(). Iterators are used to traverse over collections like lists, tuples, and dictionaries.
Q29. What is the map() function in Python?
Answer: A map() function returns an iterator (a mapping object) for each item in an iterable (such as a list). You can convert the result into a list using list(). The map() function is useful for applying operations to each item in a collection. Learning how to use map() will help you in data processing tasks.
Q30. What are the advantages of using Python?
Answer: Python is easy to learn, has a vast standard library, supports multiple programming paradigms, and is widely used in fields like web development, data science, artificial intelligence, and more. Python’s popularity in the job market makes it a great language to learn for career growth. For a structured path to mastering Python, consider taking a Python Course in Noida.
Q31. What is super() in Python?
Answer: The super() function allows you to call a method from a parent class in a child class. This is useful for extending functionality in inheritance hierarchies. Using super() properly is key to building efficient and reusable code.
Q32. What is a __call__ method in Python?
Answer: The __call__ method in Python allows an instance of a class to be called as a function. This can be useful when you want to make an object behave like a function. If you are aiming to advance your Python skills, learning about magic methods like __call__ is a must.
Q33. What is a finally block in Python?
Answer: The finally block in Python is always executed after a try block, regardless of whether an exception occurred. It’s typically used for cleanup actions, like closing files or releasing resources.
Q34. What is the __del__ method in Python?
Answer: The __del__ method in Python is a destructor that is called when an object is about to be destroyed. It’s used for cleanup operations such as closing files or network connections. To handle object destruction properly, understanding __del__ is essential.
Q35. What is the difference between a shallow copy and a deep copy?
Answer: A shallow copy creates a new object, but the elements within it still reference the same objects as the original. A deep copy creates a new object and recursively copies all the elements, including the objects referenced by the original object.
Q36. What are lambda functions in Python?
Answer: A lambda function can take any number of arguments but only has one expression. It is common to use lambda functions for quick, throwaway tasks. If you are preparing for Python coding Interview Questions and Answers, understanding how to use lambda functions is important for many coding challenges.
Q37. What is the global keyword used for in Python?
Answer: The global keyword in Python is used to declare variables that are defined outside a function, but you want to modify them inside a function. Without global, any assignment to a variable within a function creates a local variable, which can lead to confusion.
Q38. How can you handle exceptions in Python?
Answer: Exceptions in Python are handled using the try and except blocks. When an error occurs, Python jumps to the except block, where you can handle the error gracefully. You can also use finally for cleanup, regardless of whether an exception occurred.
Q39. What are Python decorators?
Answer: Decorators modify other functions or classes by changing their behavior. It is commonly used to add functionality to existing code without modifying the original function.
Q40. What is the purpose of the with statement in Python?
Answer: The with statement in Python simplifies exception handling for resource management tasks, such as opening files. It ensures that resources are properly cleaned up when the block of code is exited, even if an exception occurs. If you are preparing for Python Interview Questions for freshers, understanding with is critical when working with resources like files and network connections.
Q41. What is the yield keyword used for in Python?
Answer: A function can be turned into a generator using the yield keyword in Python. When a function contains yield, it will return an iterator that yields values one at a time instead of returning all values at once. This helps conserve memory and is useful for large datasets.
Q42. What are Python’s built-in data structures?
Answer: Python includes several built-in data structures like lists, tuples, sets, and dictionaries. Lists are mutable ordered collections, tuples are immutable ordered collections, sets are unordered collections with no duplicates, and dictionaries are unordered collections with key-value pairs.
Q43. How does Python handle memory management?
Answer: Python uses automatic memory management, including garbage collection. When objects are no longer in use, Python’s garbage collector frees up memory. It uses reference counting and cyclic garbage collection to manage memory.
Q44. What is the purpose of map() function in Python?
Answer: The map() function in Python is used to apply a given function to each item of an iterable, such as a list or tuple, and returns an iterator. It allows you to perform operations on elements in an iterable efficiently.
Q45. What is the difference between join() and split() in Python?
Answer: The join() method is used to join a sequence of strings into a single string, while the split() method splits a string into a list of substrings. Both methods are often used together when working with string manipulations. These are key concepts to understand in basic Python Interview Questions for freshers.
Q46. What is the difference between del and remove()?
Answer: The del keyword in Python removes an item at a specific index, while the remove() method removes the first occurrence of a value in a list. The difference is important when you need to delete an element from a list. Understanding these distinctions is essential for Python Interview Questions and Answers for freshers.
Q47. What is the difference between Python 2 and Python 3?
Answer: Python 3 is the latest version, and it includes improvements and fixes to many issues found in Python 2. Some differences include the print statement (Python 2 uses print "text", while Python 3 uses print("text")), integer division behavior, and Unicode support.
Q48. What is the assert statement in Python?
Answer: Asserts are used in Python to debug programs. Upon testing a condition, it raises an AssertionError if it is false. This is often used in testing and debugging.
Q49. What are Python's key features?
Answer: Some of Python's key features include its simplicity, readability, ease of integration with other languages, a large standard library, and strong community support. It is also highly portable and has extensive support for web development, data analysis, AI, and more.
Q50. How can you use regular expressions in Python?
Answer: Python’s re module allows you to work with regular expressions. Regular expressions are patterns that can be used to search for or manipulate strings. Learning regular expressions is crucial when working with data parsing, string manipulations, and text processing.
Sum up,
These top Python Interview Questions cover a wide range of topics, from basic Python programming to more advanced concepts like decorators, generators, and multithreading. Whether you are looking for basic Python Interview Questions for freshers or preparing for Python coding Interview Questions and Answers, mastering these topics will boost your chances of success in a Data Science Online Course.
Subscribe For Free Demo
Free Demo for Corporate & Online Trainings.
Your email address will not be published. Required fields are marked *
Course Features





