Building Responsive UIs With React JS And Tailwind CSS
4.9 out of 5 based on 9587 votesLast updated on 13th Nov 2024 15.9K Views
- Bookmark
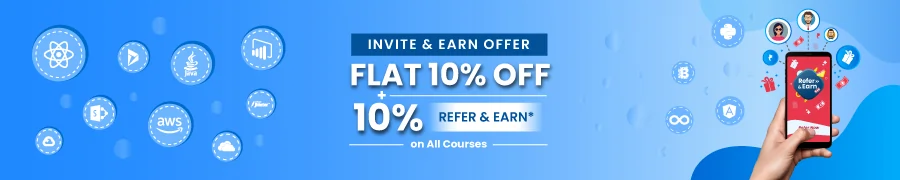
React JS and Tailwind CSS offer a powerful combo for web development, combining dynamic UI components with streamlined, responsive styling.
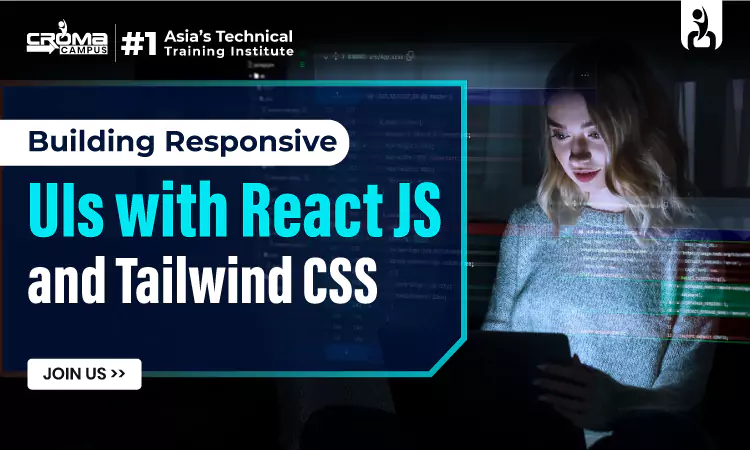
Overview
When building websites today, it’s important they look good on any device, whether it's a phone, tablet, or computer. Two tools that help you do this easily are React JS and Tailwind CSS. React is great for creating the structure of a website, while Tailwind helps you design it quickly. If you're interested in learning how to use them, getting a React JS Certification can be a great way to start.
Why React JS and Tailwind CSS?
Both React and Tailwind make web development easier. React lets you create components that handle their own content and behavior. Tailwind provides pre-made styling classes that help you design without writing much custom CSS. Together, they help you build fast, responsive websites.
If you want to learn more about these tools, consider taking a React JS Course to get deeper knowledge. If you’re interested in mobile apps, a React Native Online Course can help you learn how to use React for mobile development too.
Setting Up React and Tailwind CSS
To get started with React and Tailwind, the first step is to create a React app. You can do this with just a few commands. After that, you install Tailwind and link it to your app. Once Tailwind is set up, you can start styling your components right away. With Tailwind’s utility classes and React’s component system, you can create responsive layouts quickly and easily.
Building a Responsive Website
A responsive website looks good on any device, no matter the screen size. React and Tailwind make it easy to create these kinds of websites. React helps you create reusable components, and Tailwind makes it simple to style them for different screen sizes. Let’s break it down:
Note: If you are confused about choosing the right full-stack technology, you can go with the React Full Stack Developer Course and boost your career.
React: Creating Components
In React, everything is broken down into small pieces called components. For example, a button, navigation bar, or footer can all be components. Once you create a component, you can use it anywhere on your website. Each component can manage its own content, so you don’t need to update everything when something changes.
React makes your website more efficient. It updates only the part that needs changing, rather than reloading the whole page. This keeps the website fast.
Tailwind: Styling Your Website
Tailwind makes styling easy. Instead of writing a lot of CSS, you use classes that are already set up for you. For example, to style a button, you can use a class like bg-blue-500 for the background color and text-white for the text color. Tailwind’s utility-first approach saves you time and ensures your website has a consistent design.
Related Courses:
Mobile Application Development Online Course
Full Stack Developer Course Online
Making It Responsive
Tailwind makes it easy to adjust your design for different screen sizes. It uses breakpoints, which are markers that define when the layout should change based on the device’s size. Tailwind has breakpoints for small, medium, large, and extra-large screens. For example, you can make an element appear on small screens but hide it on larger ones, or you can change the font size on larger screens.
Here’s how it works:
Class Name | Screen Size | Effect |
sm: | 640px and above | Small screens |
md: | 768px and above | Medium screens (like tablets) |
lg: | 1024px and above | Large screens (like laptops) |
xl: | 1280px and above | Extra-large screens (desktops) |
You can add these classes to your HTML to make your website adapt to different devices.
Note: Croma Campus is one of the leading EdTech company offers React Native Certification Course online with 100% job assistance. So f you are looking for this course, do visit us now!
Example: A Simple Navbar
Let’s create a simple navigation bar that adjusts for different screen sizes. On larger screens, it will show the links horizontally. On smaller screens, the links will stack vertically, or a “Menu” button will appear.
Here’s how you can do it in React with Tailwind:
- For small screens, hide the links and show the menu button.
- On larger screens, show the links horizontally.
jsx
Copy code
import React from 'react';
function Navbar() {
return (
<nav className="bg-blue-600 p-4 flex items-center justify-between">
<div className="text-white font-bold text-xl">MyApp</div>
<div className="hidden md:flex space-x-4">
<a href="/" className="text-white">Home</a>
<a href="/about" className="text-white">About</a>
<a href="/contact" className="text-white">Contact</a>
</div>
<div className="md:hidden">
<button className="text-white">Menu</button>
</div>
</nav>
);
}
export default Navbar;
In this example, the links are hidden on small screens (using hidden), and only the "Menu" button shows. When the screen size is medium or larger (md:flex), the links become visible.
Tailwind’s Responsive Classes
Tailwind offers a simple way to design for different screen sizes. You can use the sm:, md:, lg:, and xl: classes to control how an element behaves at different screen widths. This makes it easy to design your website to look good on any device.
For example, you can add md:flex to make an element flex on medium screens and larger, or xl:text-center to center text on extra-large screens.
Also Read This:
React Full Stack Developer Salary
Mern Stack Developer Interview Questions
MEAN Stack Interview Questions
Best Practices for React and Tailwind
Using React with Tailwind is great for performance and keeping things simple. Here are some tips:
- Reusability: Use components in React to avoid repeating code. Tailwind’s utility classes can also be reused across different parts of your website.
- Purge Unused CSS: Tailwind has a purge feature that removes any unused classes in your final build. This helps keep your website fast by reducing the CSS file size.
- Avoid Complex CSS: With Tailwind, you don’t need complex CSS rules. Just use the utility classes and customize them with breakpoints.
- Keep It Simple: React and Tailwind are powerful tools that help you create clean, fast websites without too much effort. Stick to the basics and build your website piece by piece.
Performance and Speed
React and Tailwind together improve the performance of your website. React’s virtual DOM ensures that only parts of the website that need to be updated are changed. This makes your site load faster and improves its overall performance.
Tailwind’s utility-first approach helps keep your CSS file size smaller, which means quicker load times. Plus, by using Tailwind’s purge feature, you can remove any unnecessary styles in the production build, making the site even faster.
Related Full Stack Courses Online:
PHP Full Stack Developer Course
Django Full Stack Developer Course
Full Stack Dot Net Developer Course
Node JS Full Stack Developer Course
Full Stack Developer Course Python
Sum Up
With React JS and Tailwind CSS, building responsive websites is easier than ever. React helps you break down the website into small, reusable components, while Tailwind provides ready-to-use classes for styling. By using these tools, you can create websites that look great on any device and load quickly.
If you're interested in improving your skills with React, consider taking a React JS Course. You can also explore a MERN Stack Course Online to learn how to create mobile or web apps. For those interested in others like Full Stack Web Development Course, JavaScript frameworks, AngularJS Online Training can help you learn about another popular option for building websites.
Subscribe For Free Demo
Free Demo for Corporate & Online Trainings.
Your email address will not be published. Required fields are marked *
Course Features





